Through this article you will learn how to bind the record in gridview and how to delete their records on multiple checkbox select
Code behind:
Ourput: Press f5 (Debug) and see the result as follows:
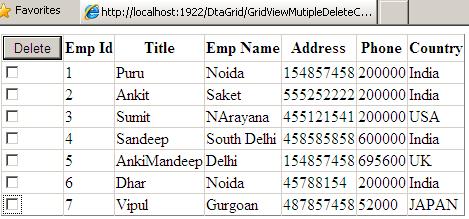
Suppose you want to delete last three records (Emp Id 5, 6, 7), first select records and click on delete button like as follows:
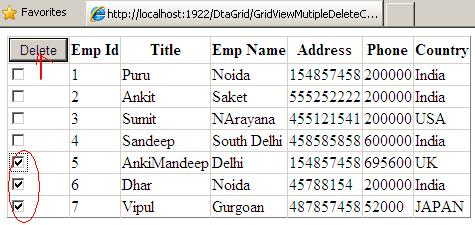
After delete you will see the remaining result as follows:
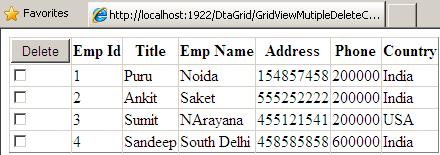
Default.aspx:
<%@ Page Language="C#" AutoEventWireup="true"CodeFile="GridViewMutipleDeleteCheckBox.aspx.cs"
Inherits="GridViewMutipleDeleteCheckBox" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN""http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script type="text/javascript" language="javascript">
function ConfirmOnDelete(item)
{
if (confirm("Would you like to delete selected item(s)?")==true)
return true;
else
return false;
}
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns=false
onrowdatabound="GridView1_RowDataBound">
<Columns>
<asp:TemplateField>
<HeaderTemplate>
<asp:Button ID="ButtonDelete" runat="server" Text="Delete"OnClick=ButtonDelete_Click/>
</HeaderTemplate>
<ItemTemplate>
<asp:CheckBox ID="CheckBox1" runat="server" />
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="EmpId" HeaderText="Emp Id"ReadOnly="True" />
<asp:BoundField DataField="EmpName" HeaderText="Title" />
<asp:BoundField DataField="Address" HeaderText="Emp Name" />
<asp:BoundField DataField="Phone" HeaderText="Address" />
<asp:BoundField DataField="Salary" HeaderText="Phone" />
<asp:BoundField DataField="Country" HeaderText="Country" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Collections.Specialized;
using System.Text;
public partial class GridViewMutipleDeleteCheckBox : System.Web.UI.Page
{
SqlConnection con = new SqlConnection();
SqlCommand cmd = new SqlCommand();
string connectionString = "Data Source=testserver;Initial Catalog=Testdatabase; uid=sa;pwd=wintellect";
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
BindGridView();
}
private void BindGridView()
{
con = new SqlConnection(connectionString);
cmd.Connection = con;
cmd.CommandText = "Select * from Puru_test_Emp";
con.Open();
GridView1.DataSource = cmd.ExecuteReader();
GridView1.DataBind();
con.Close();
}
private void DeleteRecords(StringCollection sc)
{
con = new SqlConnection(connectionString);
StringBuilder sb = new StringBuilder(string.Empty);
foreach (string item in sc)
{
const string sqlStatement = "DELETE FROM Puru_test_Emp WHERE EmpId";
sb.AppendFormat("{0}='{1}'; ", sqlStatement, item);
}
try
{
con.Open();
SqlCommand cmd = new SqlCommand(sb.ToString(), con);
cmd.CommandType = CommandType.Text;
cmd.ExecuteNonQuery();
}
catch (System.Data.SqlClient.SqlException ex)
{
string msg = "Deletion Error:";
msg += ex.Message;
throw new Exception(msg);
}
finally
{
con.Close();
}
}
protected void ButtonDelete_Click(object sender, EventArgs e)
{
StringCollection sc = new StringCollection();
string id = string.Empty;
for (int i = 0; i < GridView1.Rows.Count; i++)
{
CheckBox cb = (CheckBox)GridView1.Rows[i].Cells[0].FindControl("CheckBox1");
if (cb != null)
{
if (cb.Checked)
{
id = GridView1.Rows[i].Cells[1].Text;
sc.Add(id);
}
}
}
DeleteRecords(sc);
BindGridView();
}
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.Header)
{
Button b = (Button)e.Row.FindControl("ButtonDelete");
b.Attributes.Add("onclick", "return ConfirmOnDelete();");
}
}
}
Ourput: Press f5 (Debug) and see the result as follows:
i executed dis .. but items were not deleted
ReplyDeleteI am glad to read this. Thank you for this beautiful content, Keep it up. Techavera is the best CCNA training course in Noida. Visit us For Quality Learning.Thank you
ReplyDeleteNice article . Thank you for this beautiful content, Keep it up. Techavera is the best
ReplyDeleteEmbedded Systems training in noida
Visit us For Quality Learning.Thank you
Thanks a lot for this sharing this coding...
ReplyDeleteBEST TRAINING IN NOIDA
BEST PHP TRAINING IN NOIDA
very informative blog and useful article thank you for sharing with us , keep posting learn
ReplyDeletemore about Dot net
.NET Online Course
Thanks. Very good post.
ReplyDeleteIf you want to logo designing services than visit - Logo Designing Services Jaipur | Logo Designer in Jaipur | Logo maker in Jaipur
This comment has been removed by the author.
ReplyDeleteThe best influential discourse thoughts will be on a subject you’re keen on, aren’t overcompensated, and will be tied in with something your crowd thinks about. After you’ve picked your subject, remember these three hints when composing your influential discourse: Do your examination. Think about every one of the points. Know your crowd.
ReplyDeleteEnglish Guru offering the best English Speaking Courses in Noida.
What is a speech topic
The name Bihar is gotten from the Sanskrit and Pali word vihāra (Devanagari: विहार), signifying "homestead". The area generally including the present state was specked with Buddhist viharas, the dwelling places Buddhist priests in the old and medieval periods. Medieval author Minhaj al-Siraj Juzjani records in the Tabaqat-I Nasiri that in 1198 Bakhtiyar Khalji submitted a slaughter in a town related to the word, later known as Bihar Sharif, around 70 km away from Bodh Gaya
ReplyDeleteBihar gyan darshan
It is amazing and wonderful to visit your site.Thanks for sharing this information,this is useful.Dot Net Training in Bangalore
ReplyDeleteOur many years of experience have made us leaders in language instruction and intercultural training. We have designed programs to create world-class executives with leadership capabilities in the global context. We are best english speaking course in noida because of following reasons:
ReplyDeleteJoin English Speaking Course In Noida
Really a awesome blog for the freshers. Thanks for posting the information.
ReplyDeleteCCNA Training in Delhi
I really enjoy your blog it's a nice post
ReplyDelete.Net Online Training
Visit us: Dot Net Online Training