I will examine about the naming conventions which have to be followed while working with ASP.NET and also about Validation controls. You can either use Visual Web Developer Express Edition or Visual Studio 2005 to learn the topics covered in this article.
Naming Conventions
You have to follow certain general principles while working with every programming language. This is true with ASP.NET as well. Each data type and WebForm controls are mentioned by their respective prefixes. It would be better to follow this convention so that it would be easy to recognize the code at a later point of time. The table given below shows a list of commonly used WebForm controls and their standard prefixes.
Table - 1: Naming Conventions
WebForm Controls | Prefix | WebForm Controls | Prefix |
Button | btn | RadioButton | rad |
CheckBox
|
chk
|
RadioButtonList
|
radl
|
CheckBoxList
|
chkl
|
TextBox
|
txt
|
DataGrid
|
dgrd
|
RequiredFieldValidator
|
valr
|
DataList
|
dlst
|
CompareValidator
|
valc
|
DrpDownList
|
drop
|
RangeValidator
|
valg
|
Image
|
img
|
RegularExpressionValidator
|
vale
|
ImageButton
|
ibtn
|
CustomValidator
|
valx
|
Label
|
lbl
|
ValidationSummary
|
vals
|
LinkButton
|
lbtn
|
Table
|
tbl
|
ListBox
|
lst
|
Calendar
|
cal
|
Validation controls
One of the main tasks for every web developer is to check for errors while developing web pages. According to the server side terminology, this concept is called as Validation. Prior to ASP.NET, this task was achieved by using JavaScript and VBScript. JavaScript was commonly used by many developers and the main disadvantage of this is that developers had to write lot of code. ASP.NET simplified this task by providing us with built-in WebForm controls. With these controls you can force the user to input correct entry into the appropriate fields. You can also program in such a way that the user enters the URL in correct format. The .NET Framework 2.0 provides us with the validation controls for comparing the values between two text fields, comparing between specific ranges and a control for writing custom codes. There are six controls which are available for performing validation. They are
RequiredFieldValidator
CompareValidator
RegularExpressionValidator
RangeValidator
CustomValidator
- ValidationSummary
Let us discuss each one of them in detail
RequiredFieldValidator
This is one of the important controls used in majority of web applications. If a user fails to enter the required information on the form then the message given on this validation control will appear thereby forcing the user to fill up the required information. The code given below will illustrate this concept in detail
<p>Name: <asp:TextBox id="TextBox1" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator1" runat="server" ErrorMessage="Please enter your name" ControlToValidate="TextBox1"></asp:RequiredFieldValidator>
</p>
<p>Age: <asp:TextBox id="TextBox2" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator2" runat="server" ErrorMessage="Please enter your age" ControlToValidate="TextBox2"></asp:RequiredFieldValidator>
I have placed two textboxes and the required number of RequiredFieldValidator controls. The message given along with the ErrorMessage property will be displayed if the user doesn't enters any data on to the relevant text boxes (See the Figure given below)
Figure 1
You should set ControlToValidate property to the appropriate WebForm control in addition to usual properties such as ID. You can check out the functioning of more properties by navigating the properties box using Visual Studio 2005.
CompareValidator
With the help of this control, you can compare the value of one control with the value of another control. This control has a unique set of properties and it has been explored in the code given below
<asp:Label id="Label1" runat="server">Enter Password</asp:Label>
<asp:TextBox id="TextBox1" runat="server" TextMode="Password"></asp:TextBox>
<asp:Label id="Label2" runat="server">Enter Password again</asp:Label> <asp:TextBox id="TextBox2" runat="server" TextMode="Password"></asp:TextBox>
<asp:CompareValidator id="CompareValidator1" runat="server" ErrorMessage="Please verify password again" ControlToValidate="TextBox2" ControlToCompare="TextBox1"></asp:CompareValidator>
<asp:Button id="Button1" runat="server" Text="Submit"></asp:Button>
I have placed two textboxes and a CompareValidator control on the WebForm. The code will verify the password entered by the user and it should be equal. If it is not equal then the message inside the ErrorMessage property will be displayed. You may notice the two core properties such as ControlToValidate and ControlToCompare. The above validation control has another important property - Operator. This property has got seven values such as Equal, NotEqual, GreaterThan, GreaterThanEqual, LessThan, LessThanEqual and DataTypeCheck.
Let us now examine another usage of this control. This example will compare the two values and one of the values should be greater than the second one.
Enter First Number <asp:TextBox id="TextBox3" runat="server"></asp:TextBox>Enter Second Number <asp:TextBox id="TextBox4" runat="server"></asp:TextBox>
<asp:CompareValidator id="CompareValidator2" runat="server" ErrorMessage="First Number should be greater than the second one" ControlToValidate="TextBox4" ControlToCompare="TextBox3"Operator="GreaterThan"></asp:CompareValidator>
<asp:Button id="Button2" runat="server" Text="Button"></asp:Button>
You can use these values in a wide range of applications at different scenarios. I would suggest you to refer to the MSDN documentation for more detailed coverage regarding the usage of these property values.
RegularExpressionValidator
This control is used to verify the accuracy of strings using special characters. It will check for the specific pattern which should match with the code. You have to specify a regular expression using ValidationExpression property. The usage of this control is little complicated as compared to the above discussed controls. For example, you can verify whether a user has entered @ while inputting their e-mail address. It would be good if you have Visual Studio .NET 2003 to work with this control as it will automatically fulfill the coding part. The development environment comes with a Regular Expression Editor which will give you the corresponding code for various tasks such as expressions for Internet E-mail Address, Internet URL, US Zip Code and much more (See the figure given below)
Figure 2
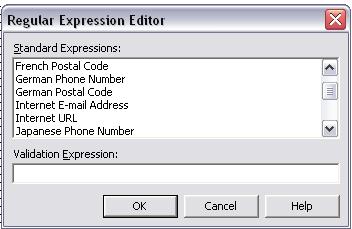
The code given below shows some of the usage of this control
Internet E-mail Address
<asp:RegularExpressionValidator id="RegularExpressionValidator1" style="Z-INDEX: 103; LEFT: 176px; POSITION: absolute; TOP: 120px" runat="server" ErrorMessage="RegularExpressionValidator"ValidationExpression="\w+([-+.]\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*"></asp:RegularExpressionValidator>
Internet URL
<asp:RegularExpressionValidator id="RegularExpressionValidator1" style="Z-INDEX: 103; LEFT: 176px; POSITION: absolute; TOP: 120px" runat="server" ErrorMessage="RegularExpressionValidator"ValidationExpression="http://([\w-]+\.)+[\w-]+(/[\w- ./?%&=]*)?"></asp:RegularExpressionValidator>
US Phone Number
<asp:RegularExpressionValidator id="RegularExpressionValidator1" style="Z-INDEX: 103; LEFT: 176px; POSITION: absolute; TOP: 120px" runat="server" ErrorMessage="RegularExpressionValidator"ValidationExpression="((\(\d{3}\) ?)|(\d{3}-))?\d{3}-\d{4}"></asp:RegularExpressionValidator>
US Social Security Number
<asp:RegularExpressionValidator id="RegularExpressionValidator1" style="Z-INDEX: 103; LEFT: 176px; POSITION: absolute; TOP: 120px" runat="server" ErrorMessage="RegularExpressionValidator"ValidationExpression="\d{3}-\d{2}-\d{4}"></asp:RegularExpressionValidator>
You may notice that the value of ValidationExpression property is little complicated and it is not at all necessary to remember these codes. Visual Studio .NET provides code for various scenarios. You can also write your own regular expressions if you have good knowledge about REGEX.
RangeValidator
With the help of this control, you can check whether users have entered a value within a specific range as specified. You can specify the range values using MinimumValue and MaximumValue properties.
<p>
<asp:Label id="Label1" runat="server">Enter your age (1-120)</asp:Label> <asp:TextBoxid="TextBox1" runat="server"></asp:TextBox>
</p>
<p>
<asp:RangeValidator id="RangeValidator1" runat="server" ErrorMessage="Please enter the correct age" ControlToValidate="TextBox1" MinimumValue="1" MaximumValue="120" Display="Dynamic"></asp:RangeValidator>
</p>
<p>
<asp:Button id="Button1" runat="server" Text="Submit"></asp:Button>
The figure given below shows the final output of the above program. You may notice that the error message will be displayed if you enter a value outside the specified range.
Figure 3
CustomValidator
The Validation controls that you have seen above are built-in within the .NET Framework. You have to apply them as such by utilizing its properties in your ASP.NET application. With the help of CustomValidator control, you can write your own validation rules and apply them on the appropriate WebForm control. A complete discussion regarding this control is beyond the scope of this article. Please refer to the MSDN documentation for additional information.
ValidationSummary
This control collects all the values from the ErrorMessage property and displays them on ASP.NET page. The data will be presented either by directly on the page or inside a MessageBox. The code given below shows the usage of this control in different ways
ValidationSummary as BulletList
Name <asp:TextBox id="TextBox1" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator1" runat="server" ErrorMessage="Enter Name"ControlToValidate="TextBox1"></asp:RequiredFieldValidator>
</p>
<p>Age <asp:TextBox id="TextBox2" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator2" runat="server" ErrorMessage="Enter Age"ControlToValidate="TextBox2"></asp:RequiredFieldValidator>
</p>
<p>
<asp:ValidationSummary id="ValidationSummary1" runat="server"></asp:ValidationSummary>
</p>
<p>
<asp:Button id="Button1" runat="server" Text="Submit"></asp:Button>
ValidationSummary as MessageBox
Name <asp:TextBox id="TextBox1" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator1" runat="server" ErrorMessage="Enter Name"ControlToValidate="TextBox1"></asp:RequiredFieldValidator>
</p>
<p>Age <asp:TextBox id="TextBox2" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator id="RequiredFieldValidator2" runat="server" ErrorMessage="Enter Age"ControlToValidate="TextBox2"></asp:RequiredFieldValidator>
</p>
<p>
<asp:ValidationSummary id="ValidationSummary1" runat="server" ShowMessageBox="True"></asp:ValidationSummary>
</p>
<p>
<asp:Button id="Button1" runat="server" Text="Submit"></asp:Button>
Summary
In this article, you have learned how to work with different validation controls included with ASP.NET 2.0. You will learn more about these controls only if you play with them.